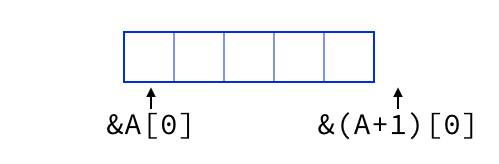
I’ve seen the following STL construct countless times:
|
|
Unless otherwise necessary, it is better to use an STL iterator because it enables you to more easily change the underlying container. You can isolate the code changes required to one line by using typedef
, as in:
|
|
Note that I wrote iter != container.end()
as opposed to iter < container.end()
. The former only requires an input iterator, while the latter requires a random access iterator—a more complicated iterator type supported by fewer STL containers.
Also note that I wrote ++iter
as opposed to iter++
. In general, you should prefer the former expression because it is always at least as efficient as the latter, and often times more so.
Of course, for a code block like the one above, you really should consider using the STL algorithm for_each
.