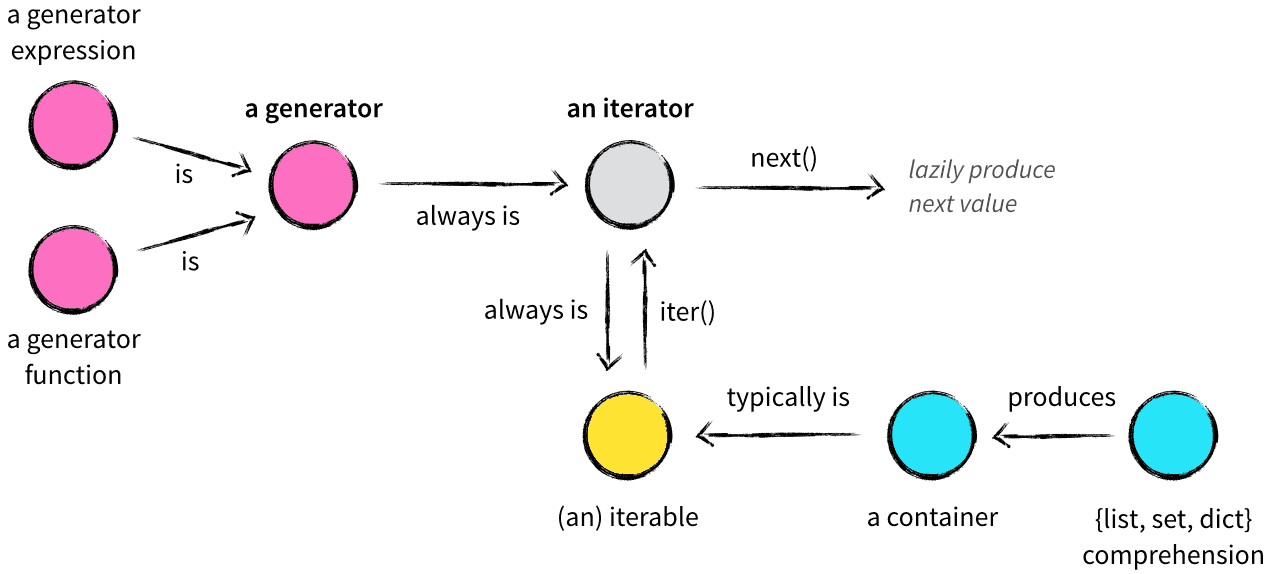
If my experience is typical, this is a very common construct:
|
|
The problem with this construct is that you have forced a container choice upon the user of your function. Slightly better, and basically your only choice when interoping with C, is this:
|
|
With the above construct you can pass in any container which uses contiguous storage, such as an array or a std::vector
(yes, std::vectors
are guaranteed to store the data contiguously). Passing a std::vector
to the above function looks like:
|
|
However, in C++ its far better to do as the STL does and write your function to accept iterators, as in:
|
|
By using this construct, you allow vast usage flexibility. Try to limit yourself to input iterator expressions on first and last (basically preincrement, dereference, and comparison) to minimize the requirements the InputIterator
class must fulfill.
Most (all?) STL containers can pass their contents to Function()
by using the containers’ begin()
and end()
functions, as in:
|
|
As C pointers are random-access iterators, you can pass arrays to Function()
as follows:
|
|
By the way, this lesson also applies to C#: prefer writing functions which accept IEnumerables
rather than collections such as arrays. (In C# 2.0, you should be able to regain the lost typesafety by accepting IEnumerable<T>
)