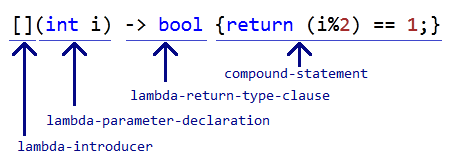
Let’s say you have a C++ function that takes a function object as a parameter and calls it:
|
|
Now let’s say you want to pass a class’s member function to call_functor()
above, as in:
|
|
The STL has a pointer-to-member function adapter called std::mem_fun()
which almost gets us there. Unfortunately, it doesn’t quite meet our needs because it requires us to pass a pointer to an instance of C, as in:
|
|
However, we can use std::mem_fun()
if we could figure out a way to create a new functor around std::mem_fun()
with &c bound as its first parameter. Unfortunately, we cannot use the STL binders (std::bind1st
and std::bind2nd
) because they only work on binary functions, not unary functions.
In the general case, you should use Boost’s very powerful binding library bind. However, let’s write our own simple binder for expository purposes.
First, we need a function, bind()
, that returns a function object which binds a parameter, p1
, to a unary function object, func
. We’ll call the returned function object binder
.
|
|
The class binder
should store func
and p1
and have an operator()
which calls func
with p1
as its parameter. For simplicity we’ll assume func
returns void:
|
|
We can now solve the initial problem by combining our bind()
with std::mem_fun()
:
|
|
We can make usage a little more convenient by introducing a macro:
|
|
There’s plenty of room for improvements, but it’s amazing what you can do with a little template trickery.