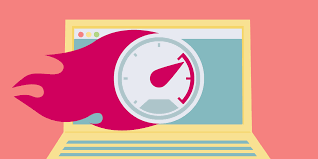
This is part 2/17 of my Exploring the .NET CoreFX series.
Thread-local storage allows you to mark a global or static variable as local to a thread. In Win32, thread-local storage is provided by the functions TlsAlloc, TlsGetValue, TlsSetValue, and TlsFree. Similarly, C# provides System.ThreadStaticAttribute and System.Threading.ThreadLocal.
Unfortunately, thread-local storage comes at a cost. Reading or writing a thread-local variable is far more expensive than reading or writing a local variable. System.Collections.Immutable uses a trick or two to help ameliorate this expense. For example, System.Collections.Immutable caches thread-local variables in local variables in a method to avoid unnecessary TLS hits on repeated access. Here’s some sample code which implements this:
|
|
Recommendations
- Minimize the use of thread-local storage. If you can, avoid it entirely.
- Minimize the number of times code accesses TLS variables. Consider caching thread-local variables in local variables in a method.