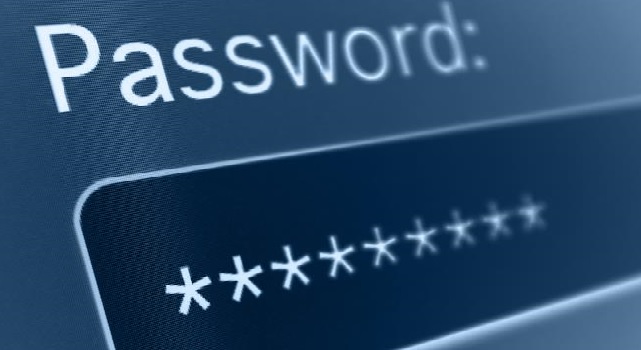
Bazel developers are currently working on adding the ability to
retrieve secrets using a credential-helper executable, similar
to how other tools like Docker and Git handle managing secrets.
Until then, the recommended approach is to store secrets in
~/.netrc
. This blog post explains how to write a custom Bazel
rule which reads secrets from ~/.netrc
.
A number of built-in Bazel rules, such as http_archive()
, natively
support retrieving credentials from ~/.netrc
. To use this feature,
you:
- Add the appropriate secret to
~/.netrc
using the typical netrc format:
machine storage.cloudprovider.com
password RANDOM-TOKEN
- Use the
auth_patterns
option inhttp_archive()
to specify how you want the secrets used:
|
|
The above causes http_archive()
to add the HTTP header
Authorization: Bearer RANDOM-TOKEN
to all outbound requests
to storage.cloudprovider.com
. This mechanism is generic enough
to handle nearly all authentication use cases.
However, let’s say you are writing your own custom rule which downloads
data over HTTP. How would you retrieve the secrets from ~/.netrc
?
You could start by reading the source code to the http_archive()
rule,
or you could just use the information below.
The key functionality is provided by the functions read_netrc()
,
read_user_netrc()
, and use_netrc()
in the file
@bazel_tools//tools/build_defs/repo:utils.bzl
. These functions parse
.netrc
files and produce the data structures necesasry to support
authentication.
A simple usage of these methods looks something like the following:
|
|
However, you are not limited to using ~/.netrc
solely for HTTP credentials – you can
use it to store basically any type of secret. I’ve also used it to store things like
GitHub auth tokens with the function below:
|
|