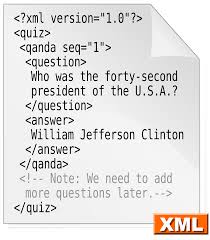
I’m not sure if this is the “canonical” way to do it but here’s a description of how to write an ASP.NET 1.1 ASPX page which returns a XML document (e.g. when writing a home-brewed web service).
First, create a new Web Form (I will call it WebService.aspx
). As we will be progamatically generating the XML in the HTTP response rather than sending the (processed) content of the ASPX file, delete everything from the ASPX file but the @Page
directive, so that it looks something like:
|
|
Next, open up the code-behind file WebService.aspx.cs
. Within the Page_Load
event handler, add the following code block:
|
|
Notice the use of the HttpResponse.OutputStream
property which allows us to write directly to the HTTP response body. Also notice that I explicitly set the Content-Type and Content-Encoding HTTP response headers, and that the encoding for both the response and the StreamWriter
must match.
Once you have this block in place, you can use whatever technique you like to write XML to the xmlWriter
object. For example, you can call XmlWriter
methods by hand, pass xmlWriter
as a parameter to XslTransform.Transform()
, or use the XmlSerializer
.