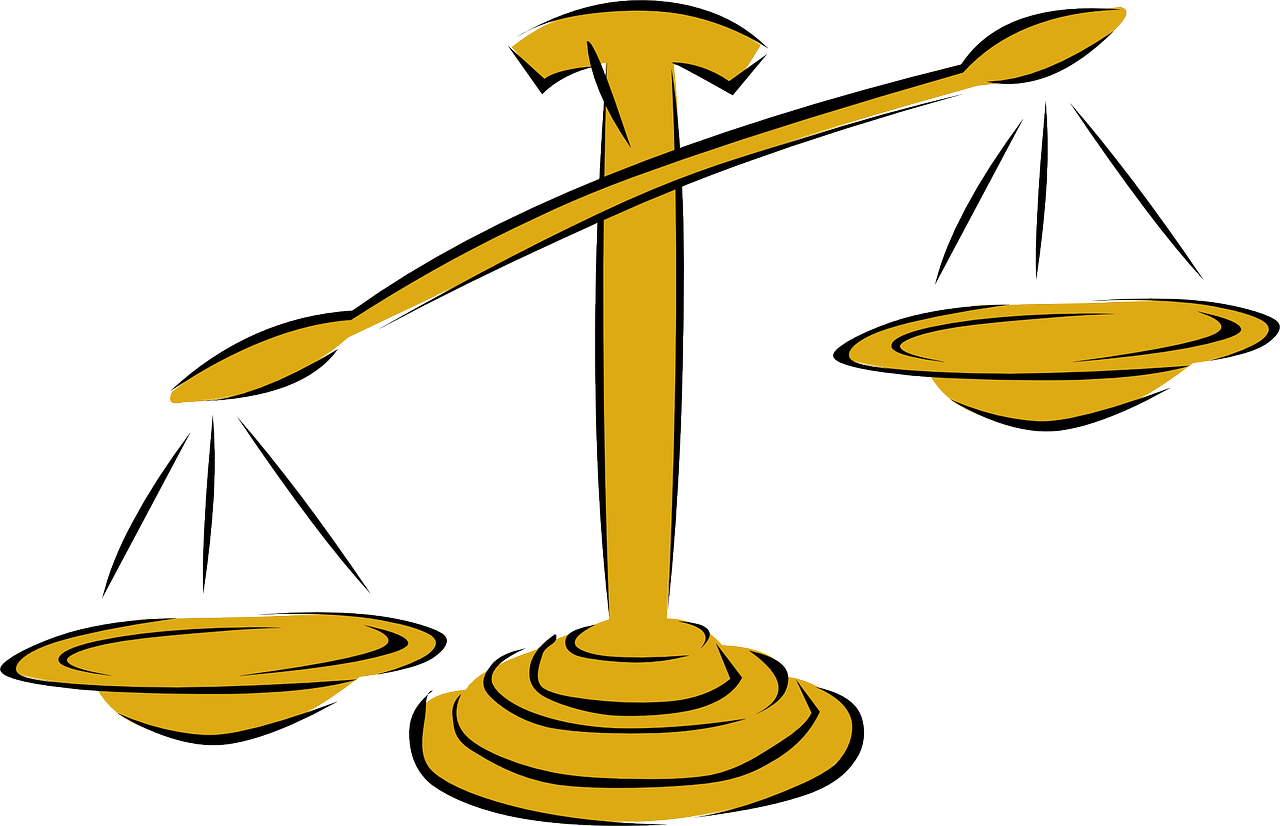
This is part 6/17 of my Exploring the .NET CoreFX series.
Let’s say you are writing a custom IList
which contains the following code:
|
|
The above code uses T
“s implementation of Object.Equals()
, which is defined as:
|
|
If T
is a value type, it will be automatically boxed by the compiler, which has a slight performance cost. However, if you knew that T
implemented IEquatable
, then you could avoid the boxing entirely. For example, this code would be slightly better performing than the above for value types:
|
|
However, it is inadvisable to require MyList
to only contain objects which implement IEquatable
.
You can get the best of both worlds by using EqualityComparer.Default
to choose IEquatable.Equals()
when available, and Object.Equals()
otherwise, as follows:
|
|
Recommendations
- Implement
IEquatable
on value types for which you expect.Equals()
to be called a lot, especially those you put into arrays, lists, or hash tables.