Exploring the .NET CoreFX Part 15: Using Non-Generic Factory Classes to Enable Type Inference
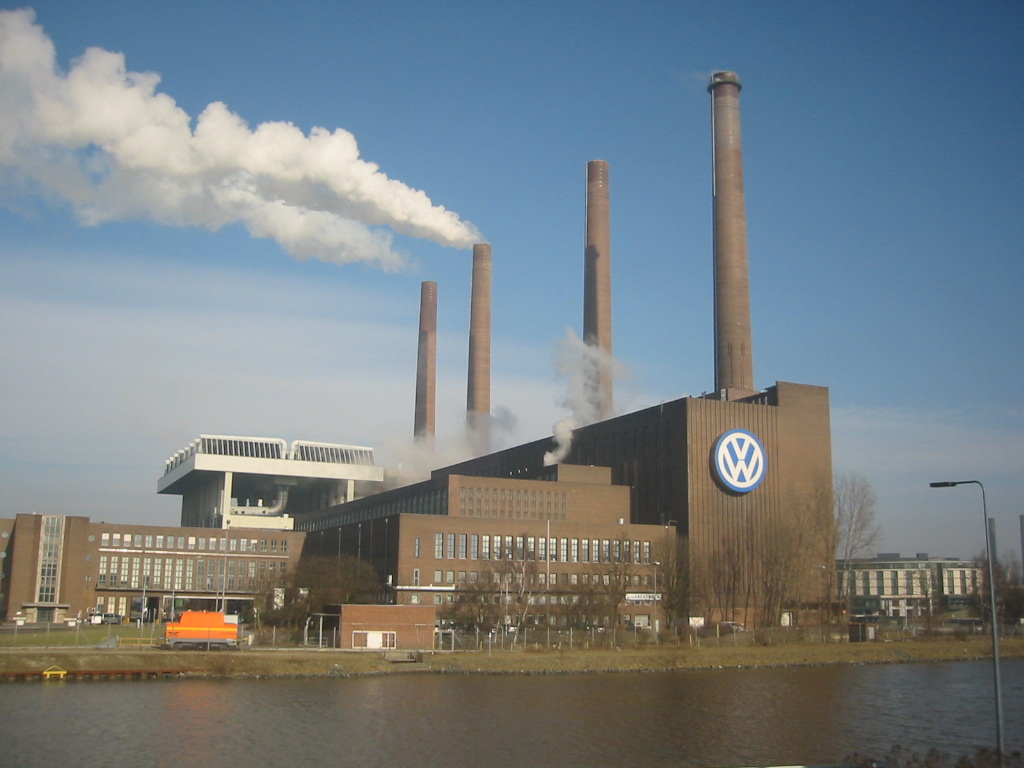
This is part 15/17 of my Exploring the .NET CoreFX series.
While C# supports type inference for generic methods, it does not support type inference for constructors. In other words, while this code works:
|
|
This code does not:
|
|
For more background on why this is, see this StackOverflow post.
Because of this, every generic type in System.Collections.Immutable includes a factory class of the same name which makes construction more convenient. For example:
|
|
The same trick is also used with System.Tuple
.
Recommendations
- If you author a generic class, consider also providing a factory class with generic methods to enable type inference.