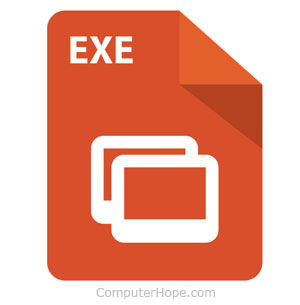
When I first started writing custom Bazel rules, I often created
separate rules for the build
and run
commands in Bazel. This
was a mistake.
For example, I once wrote rules that looked something like:
|
|
My expectation that users would bazel build //:my_app
and
bazel run //:run_app
.
This separation is silly and unnecessary. A Bazel rule can support
both build
and run
at the same time. All you have to do is declare
the rule as executable and have it return a DefaultInfo()
object with
both an executable
and a files
attribute.
For example, here is an example of a extremely simplistic cc_binary()
rule:
|
|
|
|
With this rule definition, you can generate the executable with
bazel build //:exe
and run it with bazel run //:exe
.
The above sample code can also be found at https://github.com/sengelha/practical_bazel_examples/tree/master/build_and_run_rule.