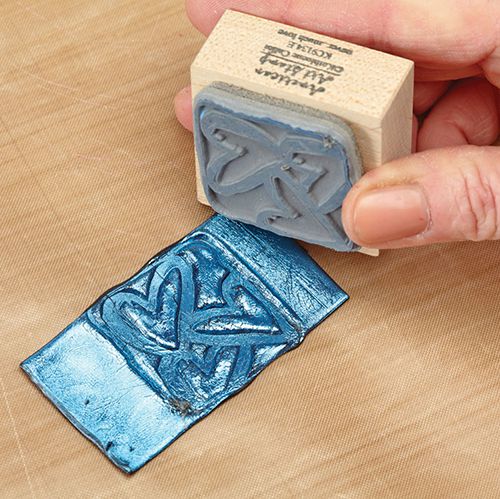
In Bazel, stamping is the process of embedding additional information into built
binaries, such as the source control revision or other workspace-related information.
Rules that support stamping typically include an integer stamp
attribute, where
1
means “always stamp”, 0
means “never stamp”, and -1
means “use the Bazel
build --stamp
flag. This blog post explains how to write a rule that supports
these values.
Quite frankly, the 0 and 1 cases are trivial; the only trick is supporting -1. We can implement this by using a combination of Bazel macros and configurable build attributes
Consider a ruleset for the hypothetical programming language mylang
.
First, define a config_setting()
that can be used to detect whether --stamp
was set on the comamnd-line:
|
|
Now, create a rule mylang_binary()
, but rather than exposing the rule directly,
wrap the rule with a macro that reads the value of private_stamp_detect
from
above:
|
|
Then implement the rule as normal, setting stamp
based on a combination of
the rule’s stamp
attribute and the value of private_stamp_detect
:
|
|
Now mylang_binary
will stamp binaries by default if users use the
bazel build --stamp
command-line optoin.